
TypeScript is a programming language that has gained significant popularity in recent years for its ability to enhance JavaScript development. It is a statically typed superset of JavaScript, meaning that it extends the capabilities of JavaScript by introducing type checking and other advanced features. In this article, we will delve into the basics of TypeScript, its evolution, key features, syntax, advantages, and how to get started with it.
Understanding the Basics of TypeScript
In order to fully appreciate the benefits of TypeScript, it is important to have a clear understanding of what it is and how it differs from JavaScript. TypeScript was first introduced by Microsoft in 2012 and has since gained a large following in the web development community. It offers a range of features and tools that can significantly improve the development process and the quality of code produced.
TypeScript is not just another programming language; it is a superset of JavaScript. This means that any valid JavaScript code is also valid TypeScript code. However, TypeScript adds additional features and syntax that JavaScript does not have, making it a more powerful and expressive language.
One of the key features of TypeScript is static typing. In JavaScript, variables can hold values of any type, and their types can change dynamically. This can lead to unexpected bugs and errors. TypeScript, on the other hand, allows developers to explicitly define the types of variables, function parameters, and return values. This helps catch errors early on and provides better tooling support. It also makes the code easier to read, understand, and maintain.
Another important feature of TypeScript is its support for object-oriented programming concepts such as classes and interfaces. JavaScript, being a prototype-based language, does not have built-in support for classes. TypeScript introduces classes, allowing developers to write more structured and modular code. Classes in TypeScript can have properties, methods, and constructors, just like in other object-oriented languages.
In addition to classes, TypeScript also supports interfaces. Interfaces define a contract that a class must adhere to. They specify the properties and methods that a class should have. This helps in achieving better code organization and reusability.
Since its initial release, TypeScript has undergone significant development and evolution. It has adopted features from the latest versions of JavaScript and has introduced its own unique features and concepts. The TypeScript team has been actively working to improve the language and make it more versatile and powerful.
TypeScript offers powerful type inference capabilities, making it possible to write code without explicitly specifying types in every instance. The compiler analyzes the code and infers the types based on the context. This reduces the amount of boilerplate code and makes the code more concise and readable.
Furthermore, TypeScript provides features like generics, decorators, and async/await syntax, which empower developers to write clean, concise, and efficient code. Generics allow developers to write reusable code that can work with different types. Decorators provide a way to add metadata and modify the behavior of classes and their members. Async/await syntax simplifies asynchronous programming, making it easier to write and understand code that deals with promises and asynchronous operations.
In conclusion, TypeScript is a powerful and versatile programming language that builds upon the foundations of JavaScript. It adds static typing, object-oriented programming concepts, and many other features that enhance the development process and improve the quality of code. Whether you are a beginner or an experienced developer, learning TypeScript can greatly benefit your web development projects.
The Syntax and Structure of TypeScript
Understanding the syntax and structure of TypeScript is crucial for writing effective code. By building on the foundations of JavaScript, TypeScript introduces several additional constructs and syntax enhancements that improve developer productivity.
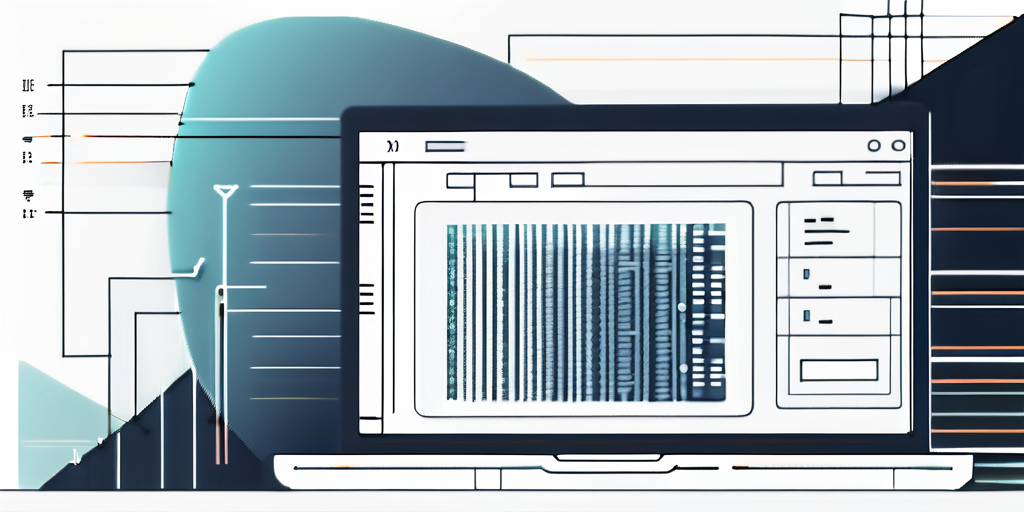
TypeScript is a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code. This makes it easy for developers to transition from JavaScript to TypeScript without much hassle. However, TypeScript goes beyond JavaScript by introducing static types, which allow variables to be explicitly typed.
Static typing is one of the key features of TypeScript that sets it apart from JavaScript. With static types, variables can have explicit types assigned to them, providing additional clarity and helping prevent bugs caused by incorrect data types. TypeScript supports various primitive types such as number
, string
, boolean
, and any
for maximum flexibility.
Basic Syntax of TypeScript
The basic syntax of TypeScript closely resembles that of JavaScript. Variables can be declared using the let
or const
keywords, and functions can be defined using the function
keyword. However, TypeScript introduces static types, allowing variables to be explicitly typed.
When declaring variables in TypeScript, you can specify the type of the variable using a colon followed by the type. For example, let age: number = 25;
declares a variable named age
with a type of number
and assigns it a value of 25
.
Furthermore, TypeScript supports type inference, which means that the type of a variable can be automatically inferred based on its initial value. This allows for more concise code while still benefiting from static typing.
Understanding TypeScript Variables
In TypeScript, variables can have explicit types assigned to them. This provides additional clarity and helps prevent bugs caused by incorrect data types. TypeScript supports various primitive types such as number
, string
, boolean
, and any
for maximum flexibility.
Additionally, TypeScript allows for the creation of custom types using interfaces and type aliases. Interfaces define the structure of an object, specifying the names and types of its properties. Type aliases, on the other hand, provide a way to create a shortcut for complex types, making the code more readable and maintainable.
By explicitly typing variables in TypeScript, you can catch type-related errors during development, reducing the chances of encountering unexpected issues in production. This leads to more robust and reliable code.
Functions and Classes in TypeScript
Functions and classes in TypeScript are similar to those in JavaScript but with added type annotations and other features. They can have explicit return types and parameter types, making it easier to understand and maintain code.
When defining functions in TypeScript, you can specify the types of the parameters and the return type using the colon notation. This provides clear documentation of the expected input and output of the function, improving code readability and maintainability.
Classes in TypeScript allow for the creation of objects with defined properties and behavior. Similar to interfaces, classes can also implement interfaces, ensuring that the class adheres to a specific contract. This promotes code reusability and modularity.
Furthermore, TypeScript introduces the concept of access modifiers, such as public
, private
, and protected
, which control the visibility and accessibility of class members. This enhances encapsulation and helps maintain the integrity of the codebase.
Overall, the syntax and structure of TypeScript build upon the familiar foundations of JavaScript, while introducing powerful features like static typing, interfaces, and access modifiers. These enhancements enable developers to write more robust and maintainable code, ultimately improving the quality and efficiency of their projects.
Advantages

There are several advantages to incorporating TypeScript into your development workflow. These advantages include enhanced code quality and readability, improved handling of large-scale projects, seamless compatibility with existing JavaScript code, and more.
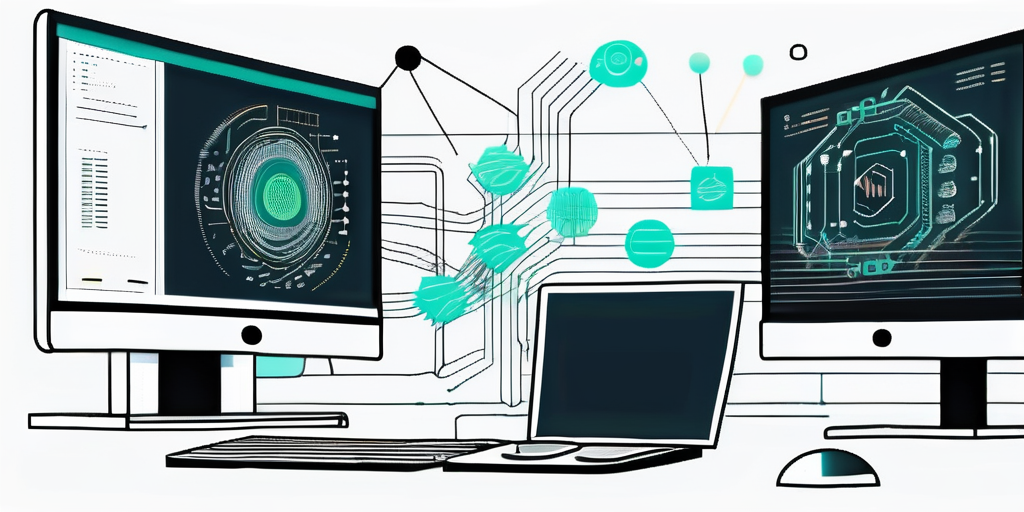
Enhanced Code Quality and Readability
TypeScript’s static typing reduces the likelihood of runtime errors, catching them during the development process itself. This leads to higher code quality and improved error detection. Additionally, explicit types make code more self-documenting and easier to understand.
With TypeScript, you can leverage features such as interfaces, classes, and modules to write more structured and maintainable code. The compiler helps enforce best practices and catches potential issues, resulting in cleaner and more readable code.
Furthermore, TypeScript supports modern ECMAScript features, allowing you to write code using the latest JavaScript syntax. This not only improves code readability but also enables you to take advantage of new language features.
TypeScript and Large-Scale Projects
Large-scale projects often involve multiple developers working on different parts of the codebase. TypeScript’s type system helps ensure that teams can effectively collaborate and understand the interfaces between different modules. This results in improved maintainability and scalability of projects.
With TypeScript, you can define clear interfaces and contracts between different components of your application. This promotes better communication and reduces the chances of integration issues. The compiler’s type checking capabilities provide early feedback, allowing developers to catch potential bugs before they become more difficult to fix.
In addition, TypeScript’s support for modules and namespaces allows you to organize your code into logical units, making it easier to navigate and maintain. This modular approach enhances the scalability of your project, as you can easily add or remove components without affecting the entire codebase.
Compatibility with JavaScript
TypeScript is fully compatible with JavaScript, allowing developers to gradually introduce it into existing codebases. This means that you can enjoy the benefits of TypeScript without having to rewrite all your code. JavaScript libraries and frameworks can be seamlessly integrated into TypeScript projects.
By incorporating TypeScript into your JavaScript project, you can leverage its static typing and other language features without sacrificing the existing functionality of your code. TypeScript’s compiler will analyze your JavaScript code and provide type annotations, enabling you to catch potential errors and improve code quality.
Furthermore, TypeScript’s compatibility with popular JavaScript frameworks, such as React and Angular, allows you to take advantage of their powerful features while enjoying the benefits of static typing. This combination enhances productivity and reduces the likelihood of runtime errors.
In conclusion, TypeScript offers numerous advantages for developers, including enhanced code quality and readability, improved handling of large-scale projects, and seamless compatibility with existing JavaScript code. By incorporating TypeScript into your development workflow, you can write cleaner, more maintainable code and build robust applications with ease.
Getting Started with TypeScript
Now that we have explored the basics of TypeScript, let’s dive into setting up your development environment and writing your first TypeScript program. This will allow you to start experiencing the power and benefits of TypeScript firsthand.
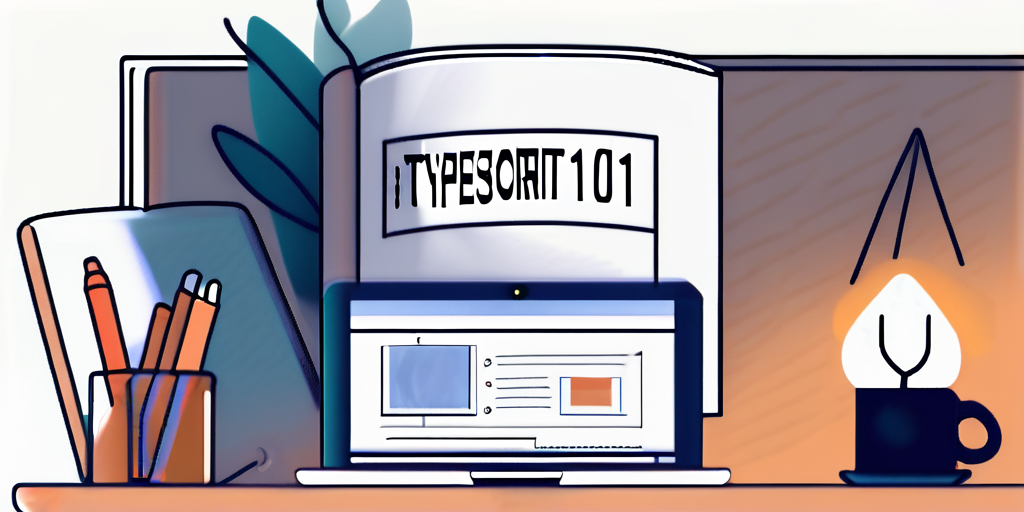
TypeScript is a superset of JavaScript that adds static typing and other features to enhance the development experience. By using TypeScript, you can catch errors early, improve code quality, and leverage advanced language features.
Setting Up Your Development Environment
Setting up a TypeScript development environment is relatively straightforward. You will need to install Node.js, which is a JavaScript runtime, and use the Node Package Manager (npm) to install TypeScript globally.
Once you have installed Node.js, open your terminal or command prompt and run the following command:
npm install -g typescript
This command will install TypeScript globally on your machine, allowing you to use it from any directory.
Additionally, you may choose to use an Integrated Development Environment (IDE) or a text editor that supports TypeScript for a more robust development experience. Some popular choices include Visual Studio Code, WebStorm, and Atom.
Writing Your First Program
Once your development environment is set up, you can start writing TypeScript code. You can create a new TypeScript file with a .ts
extension and use any text editor or IDE to open it.
Let’s write a simple TypeScript program that prints “Hello, TypeScript!” to the console:
// hello.tsconsole.log("Hello, TypeScript!");
To compile this TypeScript file into JavaScript, open your terminal or command prompt, navigate to the directory where the file is located, and run the following command:
tsc hello.ts
This command will transpile the TypeScript code into JavaScript, generating a hello.js
file.
Now, you can run the JavaScript file using Node.js:
node hello.js
You should see the output Hello, TypeScript!
in your console.
As you can see, TypeScript brings a number of advantages and features to the table. From improved code quality and readability to enhanced collaboration on large projects, TypeScript has become an essential tool for many developers. By embracing TypeScript and understanding its syntax and structure, you can take your JavaScript development to new heights.
So, what are you waiting for? Start exploring TypeScript today and unlock its full potential!