
Golang, also known as Go, is a modern programming language that has gained significant popularity in recent years. Developed by Google in 2007, Go was designed to address the challenges faced by developers in building scalable and efficient software systems. In this article, we will delve into the various aspects of Golang, exploring its origin, key features, syntax, concurrency model, standard library, and how it compares to other programming languages.
Understanding the Basics of Golang
Golang, also known as Go, was conceived by a team at Google, led by Robert Griesemer, Rob Pike, and Ken Thompson. The team aimed to overcome the limitations of existing programming languages and create a language that is easy to read, write, and maintain, while also being fast and efficient.
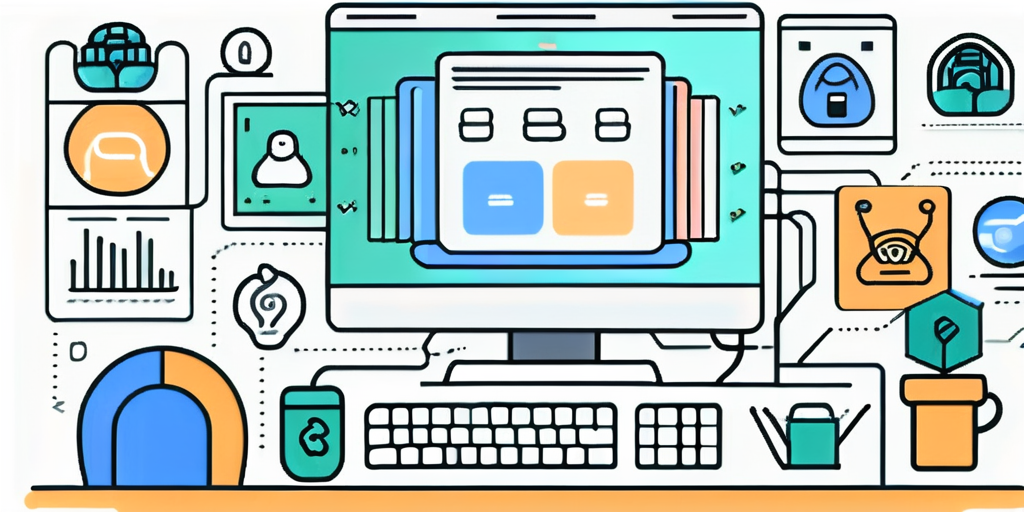
But what led to the creation of Golang? Before diving into the technical details, it’s important to understand the motivation behind its development. The team at Google observed that traditional programming languages often lacked the necessary tools and features to effectively handle modern software systems. They aimed to develop a language that would address these shortcomings and make it easier for developers to build robust and scalable applications.
One of the driving forces behind the creation of Golang was the increasing demand for concurrency. With the rise of multi-core processors and distributed systems, traditional languages struggled to handle concurrent operations efficiently. Golang aimed to provide built-in support for concurrency, making it easier for developers to write concurrent code.
The Origin and Purpose of Golang
Now let’s delve deeper into the origin and purpose of Golang. The team at Google recognized the need for a programming language that could handle the challenges posed by modern software development. They wanted a language that would be efficient, reliable, and easy to work with.
Traditional programming languages often required developers to make trade-offs between performance and ease of use. Golang aimed to bridge this gap by providing a language that combines the best of both worlds. It was designed to be fast and efficient, while also being easy to read and write.
Furthermore, Golang was created with the goal of simplifying concurrent programming. Concurrency is a key aspect of modern software systems, and Golang aimed to make it easier for developers to handle concurrent operations. By providing built-in support for concurrency, Golang reduced the complexity associated with writing concurrent code.
Key Features of Golang
Golang incorporates several innovative features that set it apart from other programming languages. Firstly, its static type system ensures better code reliability and performance. By checking types at compile-time, Golang catches many errors before the code is even run, leading to more robust and efficient programs.
In addition to its static type system, Golang’s garbage collection capabilities make it easier to manage memory. Memory management is a critical aspect of programming, and Golang’s garbage collector automatically frees up memory that is no longer in use. This reduces the risk of memory leaks and crashes, making Golang a more reliable choice for building software.
Another notable feature of Golang is its simple and intuitive syntax. The language was designed to be easy to read and write, with a focus on minimizing the cognitive load on developers. This simplicity extends to Golang’s approach to error handling as well. Golang encourages explicit and concise error checking, making it easier for developers to handle errors effectively.
Overall, Golang offers a powerful and efficient programming language that addresses the limitations of traditional languages. With its focus on simplicity, concurrency support, and robust features, Golang is becoming increasingly popular among developers for building modern software systems.
Diving Deeper into Golang’s Syntax
Understanding Golang’s syntax is crucial to effectively write code in the language. Let’s explore some key aspects of Golang’s syntax.
Golang, also known as Go, is a statically typed programming language that was developed at Google. It was designed to be simple, efficient, and easy to read. One of the main goals of Go’s syntax is to reduce clutter and make code more concise.
One of the key features of Golang’s syntax is its strong type system. In Go, variables must be declared with a specific type before they can be used. This approach helps catch errors at compile-time and improves program reliability. For example, if you try to assign a string value to an integer variable, the compiler will throw an error.
Golang also supports various data types, such as integers, floats, booleans, strings, and more. The language provides a rich set of built-in functions and operators to manipulate and work with these types. For example, you can use the “+” operator to concatenate strings or perform arithmetic operations on numbers.
Control Structures and Functions in Golang
Golang offers familiar control structures like if-else statements, for loops, and switch statements. These structures allow you to control the flow of your program based on certain conditions. For example, you can use an if-else statement to execute a block of code if a certain condition is true, or execute a different block of code if the condition is false.
One interesting feature of Golang’s control structures is the ability to handle multiple variables in a for loop or switch statement. This can be useful when you need to iterate over multiple collections or perform different actions based on multiple conditions.
Functions in Golang are integral building blocks of code organization. They allow for code modularity and can be reused across different parts of a program. In Go, functions are defined using the “func” keyword, followed by the function name, parameters, and return type (if any). For example, you can define a function that takes two integers as parameters and returns their sum.
Golang also supports the concept of anonymous functions, known as closures. A closure is a function that is defined inside another function and has access to the variables in its parent function. This provides flexibility in handling different scenarios and can be particularly useful when working with concurrency or asynchronous programming.
In conclusion, Golang’s syntax is designed to be simple, efficient, and easy to read. Its strong type system, support for various data types, familiar control structures, and powerful functions make it a versatile language for writing clean and reliable code.
Golang’s Concurrency Model

One of the standout features of Golang is its built-in support for concurrency. Let’s explore how Golang handles concurrency and the key concepts involved.
Golang’s concurrency model revolves around the use of Goroutines and Channels, which provide a powerful and efficient way to handle concurrent tasks.
Goroutines and Channels
Goroutines in Golang are lightweight threads that allow for concurrent execution of code. They provide a simple and efficient way to handle concurrent tasks. Unlike traditional thread-based concurrency models, Goroutines in Golang are managed by the Go runtime, making them more efficient and scalable.
When a Goroutine is created, it is scheduled to run independently of other Goroutines, allowing for parallel execution of code. This parallelism is achieved without the need for explicit thread management or synchronization primitives.
Channels, on the other hand, enable safe communication and synchronization between Goroutines. They act as a conduit for data exchange, ensuring that Goroutines can communicate without directly accessing shared variables. Channels provide a powerful mechanism to build concurrent systems that are free from race conditions and other synchronization issues.
By using channels, Goroutines can send and receive data to and from each other, allowing for seamless coordination and collaboration. This communication is done through channel operations, which block until a sender and receiver are both ready, ensuring that data is safely exchanged.
Select Statement in Golang
The select statement in Golang allows for non-blocking communication between Goroutines. It enables Goroutines to wait for multiple channel operations simultaneously and proceed with any operation that becomes ready first. This feature is particularly useful when dealing with multiple channels and Goroutines that need to communicate efficiently.
The select statement works by evaluating a set of channel operations and selecting the one that is ready to proceed. If multiple operations are ready, one is chosen at random. This allows for Goroutines to efficiently handle multiple channels without blocking unnecessarily.
With the select statement, developers can build highly responsive and efficient concurrent systems that can handle multiple inputs and outputs concurrently. It provides a powerful tool for managing complex communication patterns between Goroutines.
In conclusion, Golang’s concurrency model, based on Goroutines and Channels, provides a powerful and efficient way to handle concurrent tasks. By using lightweight threads and safe communication mechanisms, Golang enables developers to build highly scalable and responsive concurrent systems. The select statement further enhances the capabilities of Golang’s concurrency model, allowing for efficient non-blocking communication between Goroutines.
Golang’s Standard Library
Golang’s standard library offers a wide range of packages that provide a wealth of functionality to developers. Let’s take a closer look at some commonly used packages.
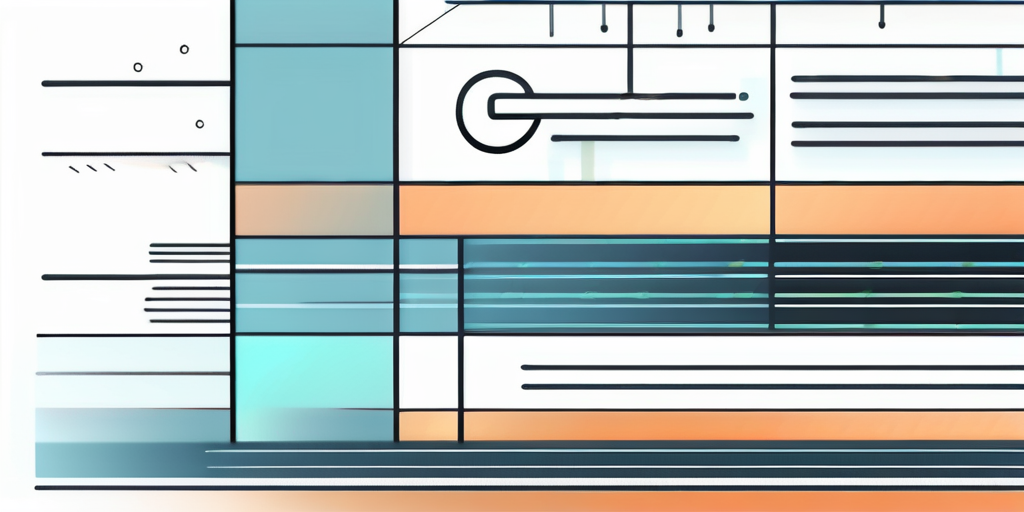
The fmt
package is one of the most frequently used packages in Golang. It provides functions for formatted input/output operations, making it easy to display and manipulate data on the console.
With the fmt
package, developers can easily format strings, numbers, and other data types. It offers a variety of formatting options, such as specifying the width and precision of numbers, aligning text, and adding leading zeros. This package also provides functions for reading input from the console, allowing developers to interact with users and build interactive command-line applications.
The net/http
package is another notable package, offering a comprehensive set of tools for building web applications. It enables developers to handle HTTP requests and responses, create web servers, and interact with external APIs.
With the net/http
package, developers can easily create HTTP servers that listen for incoming requests and respond with appropriate content. They can define routes to handle different URLs, parse request parameters, and handle file uploads. This package also provides functions for making HTTP requests to external APIs, allowing developers to integrate their applications with other web services.
File Handling in Golang
Golang provides robust support for working with files and directories. The os
package offers functions for manipulating files, creating directories, and managing file permissions. This powerful file handling capability allows developers to efficiently manage file I/O operations in their programs.
With the os
package, developers can easily create, open, read, write, and delete files. They can also retrieve information about files, such as their size, modification time, and permissions. This package provides functions for navigating directories, listing files in a directory, and recursively traversing directory trees. Developers can also set file permissions, change ownership, and perform other file-related operations.
In addition to the os
package, Golang also provides other packages for specific file handling tasks. For example, the path/filepath
package offers functions for manipulating file paths, resolving relative paths, and working with file extensions. The io/ioutil
package provides convenient functions for reading and writing files, handling file buffers, and performing file-related operations in a more streamlined manner.
Comparing Golang with Other Languages
While Golang has gained significant popularity, it’s essential to understand how it compares to other programming languages. Let’s explore a few comparisons to get a better perspective.
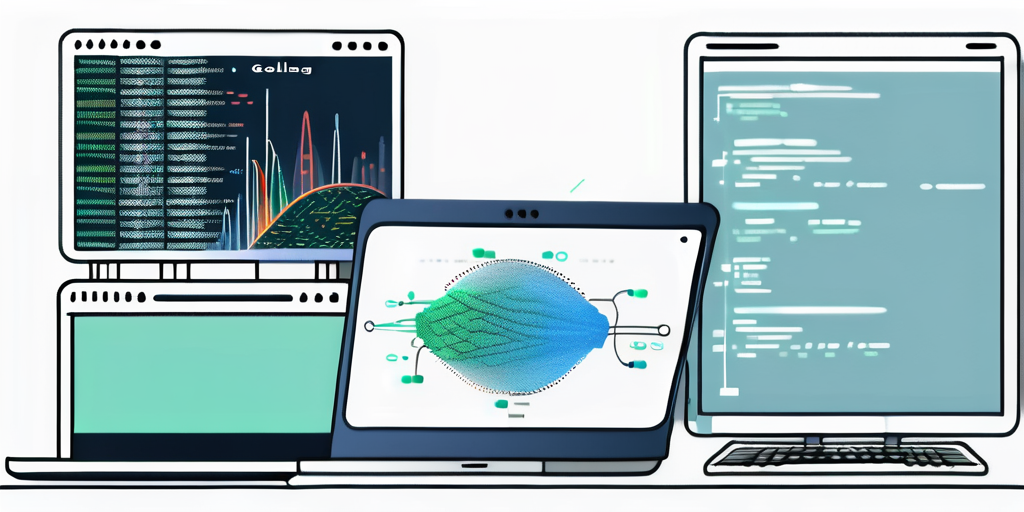
When comparing Golang with Python, it becomes evident that both languages have their strengths and weaknesses. Golang shines in terms of performance and concurrency, making it a popular choice for building high-performance systems. Its compiled nature allows Golang to execute code faster than interpreted languages like Python. Additionally, Golang’s built-in concurrency support, through goroutines and channels, enables developers to write highly concurrent and efficient programs. On the other hand, Python’s ease of use and extensive ecosystem make it a preferred language for rapid development and data analysis. Python’s syntax is known for its simplicity and readability, making it an excellent choice for beginners and experienced developers alike. Moreover, Python’s vast collection of libraries and frameworks, such as NumPy, Pandas, and Django, provide developers with powerful tools for data manipulation, scientific computing, and web development.
Conclusion
Now, let’s compare Golang with Java. These two languages cater to different use cases and have different strengths and weaknesses. Java’s vast ecosystem, mature tooling, and extensive library support make it a reliable choice for enterprise-level applications. Java’s object-oriented nature and strong static typing ensure robustness and maintainability in large-scale projects. Additionally, the Java Virtual Machine (JVM) allows Java programs to run on any platform, making it highly portable. On the other hand, Golang, with its simplicity and built-in concurrency support, is better suited for building scalable and performant systems. Golang’s static typing and strict error checking help catch bugs at compile-time, reducing the chances of runtime errors. Furthermore, Golang’s garbage collector efficiently manages memory, ensuring optimal performance even in highly concurrent applications.
In conclusion, Golang is a revolutionary programming language that simplifies the development of robust and scalable software systems. With its unique features, including built-in concurrency support, intuitive syntax, and a powerful standard library, Golang provides developers with a versatile toolset for tackling modern software challenges. Whether you are a seasoned developer or just starting your programming journey, Golang is definitely a language worth exploring.